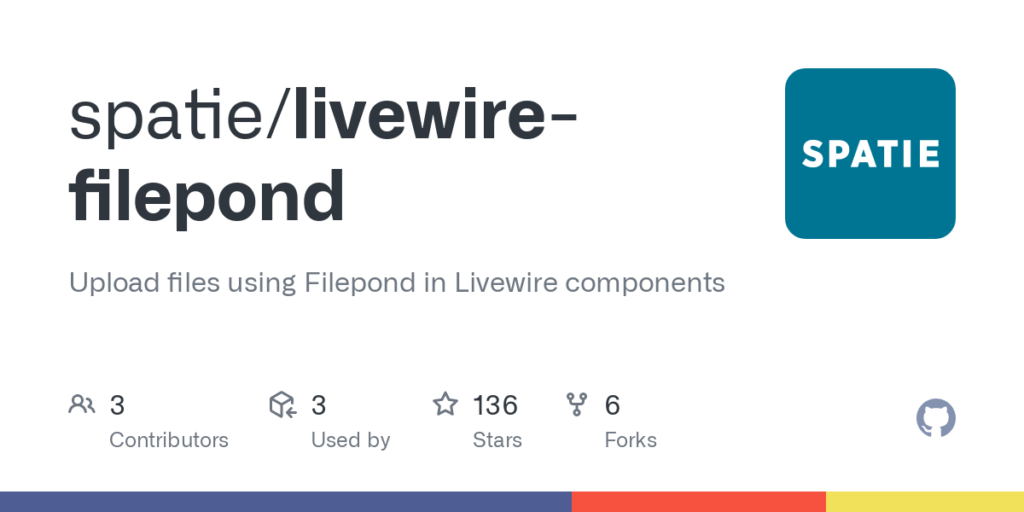
Uploading files in web applications can be made seamless and efficient with a versatile JavaScript package known for its powerful features. This tool offers seamless integration with popular frameworks like React, Vue, and Svelte, making it a top choice for developers. If you’re working with Livewire in Laravel, you can now integrate this package into your projects using Spatie’s livewire-filepond package.
What is FilePond?
Robust JavaScript library designed to handle file uploads with ease. It offers a user-friendly interface and supports many features such as drag-and-drop, image previews, and asynchronous uploads. Its compatibility with various JavaScript frameworks makes it a versatile tool for web developers.
Integrating FilePond with Livewire
Livewire is a full-stack framework for Laravel that simplifies the creation of dynamic interfaces. By leveraging spatie’s livewire-filepond package
Key Features of Livewire FilePond
- Drag and Drop: Easily drag files into the upload area.
- Instant Uploads: Upload files as soon as they are selected or dropped.
- Image Previews: View image previews before uploading.
- Chunked Uploads: Efficiently handle large file uploads by splitting them into smaller chunks.
- Asynchronous Uploads: Improve performance with asynchronous file uploads.
How to Set Up
Step 1: Install
Start by installing Using Composer and npm:
composer require livewire/livewire
npm install filepond --save
Step 2: Configure
Add the necessary JavaScript and CSS files to your Blade templates:
<link href="https://unpkg.com/filepond/dist/filepond.css" rel="stylesheet">
<script src="https://unpkg.com/filepond/dist/filepond.js"></script>
Step 3: Create a Livewire Component
Generate a component to handle file uploads:
php artisan make:livewire FileUpload
Step 4: Implement File Upload Logic
In the component class, add the file upload logic:
use Livewire\Component;
use Livewire\WithFileUploads;
class FileUpload extends Component
{
use WithFileUploads;
public $files = [];
public function save()
{
foreach ($this->files as $file) {
$file->store('uploads');
}
}
public function render()
{
return view('livewire.file-upload');
}
}
Step 5: Create the Blade View
Include the input field and bind it to the component:
<div>
<input type="file" wire:model="files" multiple>
<button wire:click="save">Upload</button>
</div>
Step 6: Enhance
Enhance the file input:
<script>
FilePond.create(document.querySelector('input[type="file"]'));
</script>
Conclusion
By following the steps outlined above, you can enhance your application’s file upload functionality, providing a better user experience.
For additional resources and tutorials on Laravel and Livewire, check out the following links: